Managing tasks efficiently is the backbone of any successful project. Developers and teams constantly seek tools that can streamline workflows, enhance state management, and ensure scalability. One such powerful solution is @ngrx/signalstore, a state management library that takes reactivity and performance to the next level. But how does it truly transform task management? Let’s explore its capabilities, benefits, and real-world applications.
What is @ngrx/signalstore?
@ngrx/signalstore is a lightweight, reactive state management library designed for Angular applications. It leverages Angular Signals, a feature that enhances change detection and reactivity while minimizing performance overhead. Unlike traditional state management solutions, @ngrx/signalstore provides a more streamlined, declarative approach that simplifies complex state logic.
Why Use @ngrx/signalstore for Task Management?
Task management requires efficient state handling, real-time updates, and a seamless user experience. @ngrx/signalstore addresses these needs by offering:

- Improved Performance: Signals eliminate unnecessary re-renders, ensuring a smooth experience.
- Simplified State Handling: Reduces boilerplate code, making state management more intuitive.
- Reactive Updates: Automatically reacts to changes, keeping task lists synchronized.
- Minimal Learning Curve: Provides a straightforward API, making it easier to adopt.
How Does @ngrx/signalstore Work?
Understanding how @ngrx/signalstore operates can help in leveraging its full potential. It primarily consists of:
1. Defining a Store
Stores are central to managing application state. With @ngrx/signalstore, creating a store is simple:
typescriptCopyEditimport { signalStore, withState } from '@ngrx/signalstore';
export const TaskStore = signalStore(
{
name: 'tasks',
withState<{ tasks: string[] }>({ tasks: [] })
}
);
2. Updating State
State modifications are done through immutable updates, ensuring predictable state changes.
typescriptCopyEditimport { withMethods } from '@ngrx/signalstore';
export const TaskStore = signalStore(
{ name: 'tasks', withState<{ tasks: string[] }>({ tasks: [] }) },
withMethods((store) => ({
addTask: (task: string) => store.update((state) => ({ tasks: [...state.tasks, task] })),
removeTask: (task: string) => store.update((state) => ({ tasks: state.tasks.filter(t => t !== task) }))
}))
);
3. Accessing the Store in Components
Accessing and displaying tasks in Angular components is seamless.
typescriptCopyEdit@Component({
selector: 'app-task-list',
template: `
<ul>
<li *ngFor="let task of taskStore.tasks()">{{ task }}</li>
</ul>
`,
})
export class TaskListComponent {
taskStore = inject(TaskStore);
}
Comparing @ngrx/signalstore with Traditional State Management
Feature | @ngrx/signalstore | NgRx (Redux-based) | Services with BehaviorSubject |
---|---|---|---|
Reactivity | High | Medium | High |
Boilerplate Code | Minimal | Extensive | Moderate |
Performance | Optimized | Can be heavy | Lightweight |
Ease of Use | Easy | Steep learning curve | Moderate |
Benefits of Using @ngrx/signalstore in Task Management
1. Real-Time Synchronization
Tasks update instantly across components without unnecessary re-rendering, making applications more efficient.
2. Enhanced Code Maintainability
With minimal code complexity, developers can focus on building features instead of managing state.
3. Scalability for Large Applications
Handles large-scale task management without performance degradation.
4. Seamless Integration with Angular Ecosystem
Works well with Angular’s built-in features, making it an ideal choice for Angular applications.
Is @ngrx/signalstore the Right Choice for Your Project?
If your project demands a reactive, efficient, and easy-to-use state management solution, then @ngrx/signalstore is a game-changer. It eliminates unnecessary complexities, enhances performance, and ensures a smooth development experience.
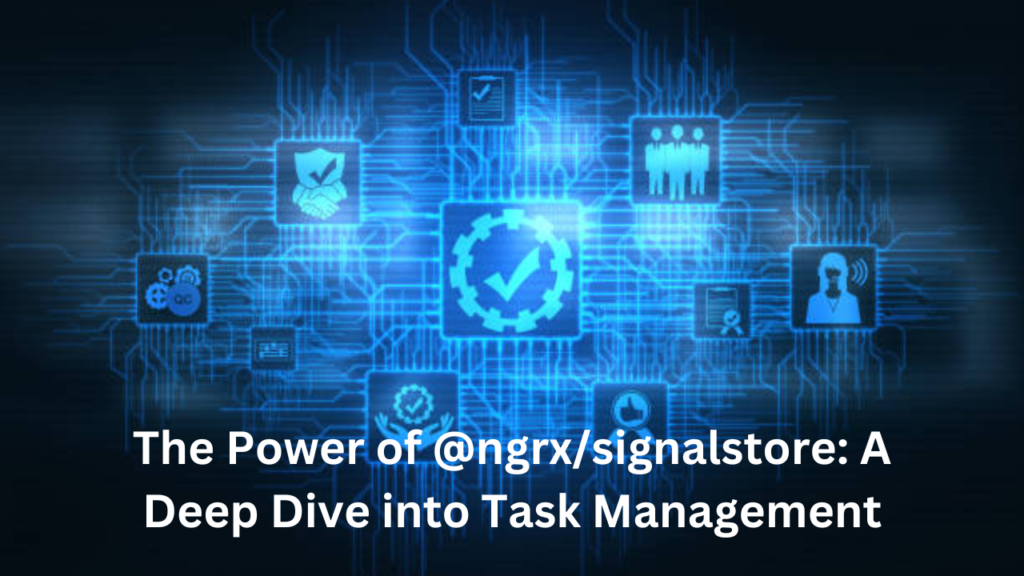
Also Read: Online PlayMyWorld – A Fun and Engaging Gaming Experience
Final Thoughts
The world of state management is evolving, and @ngrx/signalstore is at the forefront of this transformation. Its power in task management makes it a must-have tool for developers seeking efficiency and scalability. By leveraging its capabilities, teams can build responsive, high-performing applications with ease.
Are you ready to supercharge your task management workflow? @ngrx/signalstore might just be the perfect solution for your next Angular project.
Comments 1